Definition of Applet in Java :
- An Applet in Java is a system-defined/in-built/pre-defined class present inside an applet package which is used to develop a client-side java program and is executed with the help of any web browser and finally gives quick results.
-
In other words, it is a special type of Java program that is embedded inside HTML code/page in the form of a web page to generate the dynamic output. It runs inside the web browser of the client computer.
-
An Applet is normally a small Java program that can be embedded into a web page i.e. an Applet program is normally embedded with an HTML page using the Applet/Object tag and finally the page is hosted on a web server as a web document with the help of internet/online connection so that page is available/distributed widely all over the world.
- An Applet is a small program that is intended not to be run on its own, but rather to be embedded inside another application.
Features/Characteristics of Applet in Java :
- Class name of applet is=Applet; package name of applet is=applet; To import applet package in a java program to create new user-defined applet class is=import java.applet.Applet or import java.applet.*
- A Java Virtual Machine (JVM) is required to view an applet output. The JVM can use either an available plug-in of the Web browser or a separate runtime environment (applet viewer) to run an applet program.
- An Applet program does not contain the main () method in its program.
- The Applet class provides a standard interface between Applets and their environment.
- It is a secure program that runs inside a web browser.
- The applet can be embedded in an HTML page. Applets differ from Java applications in the way that they are not allowed to access certain resources on the local computer, such as files and serial devices (modems, printers, etc.), and are prohibited from communicating with most other computers across a network.
- The common rule is that an Applet can only make an Internet connection to the computer from which the Applet was sent.
- An output of an applet program has appeared as a graphical user interface (GUI) form.
- Applet programs require limited access to resources during processing so it can run even complex computations easily and safely.
- The JVM on the user’s machine creates an instance of the applet class and invokes various methods during the applet’s lifetime.
- Applets have very high security.
- Typically each Applet program must import two packages – java.awt.Component and java.applet.Applet.
- Here java.awt.Component imports the Abstract Window Toolkit (AWT) classes. The Applets program interacts with the user (either directly or indirectly) via the AWT controls. The AWT class contains features of window-based, graphical user interface whereas java.applet.Applet imports the applet package, which contains the class Applet which supplies major life cycle methods as well as the standard interface between the applet and the browser environment.
- Each applet program that is created by the user(user applet program) is really a part of the Applet class i.e. that created class is actually a subclass or derived class of the Applet class and is created by using extends
- The user-created applet program/subclass must be declared as public, to access by others easily.
- Each Applet program must contain a paint() method to display/print its output.
- To use Swing GUI components in an Applet program, a special class of Swing is used called jApplet and is included as import javax.swing.jApplet.
- JVM creates an instance of the applet class and invokes init() method first to initialize an Applet.
Advantage
- Applet applications are used to design dynamic web page and distributed program.
- Applet programs are easy to develop just by extending the Applet class mainly.
- Since it runs on the client’s browser hence it takes very less time to execute the applet program.
- Applets program are highly secured.
- It can be executed by any web browser easily running under any platforms (operating systems) such as Linux, Windows, Mac but not on android operating system.
Disadvantage
- To run an applet program successfully, certain Java plugs-in (responsible to manage the life cycle of an applet) are required to install.
- Android operating system does not support/run Java applet program.
- Some applet programs require a specific framework or environment called Java Runtime Environment (JRE), to run it successfully.
Life Cycle of Applets
- There are two classes required to use all the methods of applet life cycle. They are java.applet.Applet class which provides four major methods (init(), start(), stop(), destroy()) and java.awt.Component class which provides single method (paint()) for an applet life cycle.
- When the Applet program is executed from the Appletviewer, The Appletviewer only understands the <Applet> and </Applet> HTML tags, so it is sometimes referred to as the “minimal browser”.(It ignores all other HTML tags). The starting sequence of method calls made by the Appletviewer or browser for every Applet is always init (), start () and paint () – this provides a start-up
sequence of method calls as every Applet begins execution.
Stages of Applet Life Cycle
The life cycle of Applet is completed in five stages/steps using related specific methods:-
[a] New/Initialized
[b] Start/Started
[c] Running/Painted
[d] Idle/Stopped
[e] Destroy/Destroyed
Applet Life Cycle Methods
The main function of five Applet life cycle methods are –
[i] init() :
This method is called first and only once during its life cycle to initialize the applet program when an applet program is going to load.
[ii] start() :
This method is called after init() when the applet program starts running and also restart certain steps of applet cycle again after it has been paused/stopped due to certain reason. It runs each time when html page is displayed on the screen.
[iii] paint() :
This method is immediately called after the start method and also any time the applet needs to repaint itself in the browser i.e. when the applet begins execution or whenever the applet must redraw its output.
The paint( ) method has single parameter of class type named Graphics with its object (g). This parameter contains all the related graphics context which describes a required complete graphics environment in which the applet is running. This environment is used whenever output to the applet is required. This method provides Graphics class object that can be used for drawing oval, rectangle, arc etc. shape easily.
[iv] stop() :
This method is used to suspend or pause applet program that does not need to run or if browser is in minimized state. This method is always called before destroy () method. We can restart the suspended part of the program if the user back to the previous working page again then start( ) is called automatically.
[v] destroy() :
This method is called when an applet program/browser is going to close permanently. It is also called only once but in the last situation of the program. This method helps to release all the used resources.
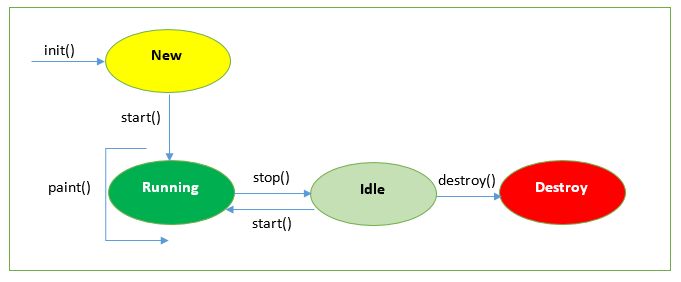
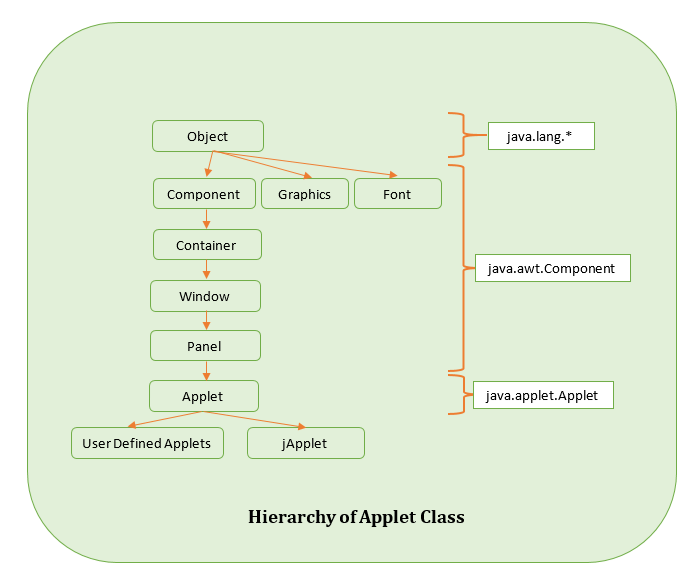
Syntax
public class classname extends Applet
{
……
…… (source codes of required lifecycle methods in proper way)
……
}
An Applet program can be run in two ways –
[A] Using java supported/enabled/compatible web browser (using html page)
- The supported Web browsers for Applets are Netscape Communicator, Microsoft Internet Explorer etc.
- In the Appletviewer, we can execute an Applet program again by clicking the Applet viewer’s Applet menu and selecting the Reload option from the menu. To terminate an Applet, click the Appletviewer’s Applet menu and select the Quit option.
- Html support a predefined tag called <applet> which helps to load the applet program on the web browser window.
- Here class defined must be public because object created by Java Plug-in software during run time resides on the web browser.
Steps to Create Applet Program using HTML Page are:-
Step1:
To execute the applet program with the help of html file, we first of all create an java applet program and save it (say as emp.java) and only compile it (say as javac emp.java) and make it error free (not necessary to interpret it).
import java.applet.Applet;
import java.awt.Graphics;
public class emp extends Applet
{
public void paint(Graphics g)
{
g.drawString(“Welcome Codershelpline”,500,500);
}
}
Save as = emp.java
compile as = javac emp.java
Not interpret it as=java emp
Step2:-
Now we create an html file (and save this html file (as say as emp1.html) in the same folder/directory where class file is created/present) and write java class file name (say emp.class) inside applet tag.
<html>
<body>
<applet code=”emp.class” width=”150″ height=”150″> </applet>
</body>
</html>
Save as file = emp1.html
Run as = by simply double clicking emp1.html file
Output=Welocme Codershelpline
(in an appletviewer window having size or width 500 and height 500 and output is displayed inside the applet window at 150-150 distance from X and Y axis).
Step3:-
Now store both the files (say emp.java and emp1.html) into the same directory or folder in any drive. Now run the html file (emp1.html) [by simply open or click it or double click it] to display the output.
NB: we can also run web browser or html file created applet program using appletviewer tool.
Save first file as = emp.java
compile first file as = javac emp.java
Save second file as = emp1.html(store first and second file in same folder or directory or drive)
Run as = (write at any command prompt say d:\) appletviewer emp1.html
Output=Welcome Codershelpline (result displays in a appletviewer window, having size or width 500 and height 500 and output is displayed inside the applet window at 150 150 distance from X and Y axis)
[B] Using applet viewer tool (with Java program)
- Since some web browsers are not java compatible/enable and hence they do not support <applet> tag used in the applet program, so Sun Microsystem was introduced a special standard tool to run applet program easily without use of web browser is called applet viewer. This program is run inside an applet viewer window. This is the easiest way to run an applet program.
- To run program in applet viewer, An Applet program must contain <applet> tag which is written inside an html as comment line so that applet viewer tools can identify and run the applet program easily.
import java.applet.Applet;
import java.awt.Graphics;
/*<applet code=”emp2.class” width=”500″ height=”500″></applet>*/
public class emp2 extends Applet
{
public void paint(Graphics g)
{
g.drawString(“Hello Codershelpline”,150,150);
}
}
Save file as = emp2.java (in a folder or a drive)
Compile as = javac emp2.java (No need to interpret)
Run as = (write at any command prompt say C:\) appletviewer emp2.java
Output=Hello Codershelpline (in a appletviewer window having size or width 500 and height 500 and output is displayed inside the applet window at 150-150 distance from X and Y axis)
1 Comment
Krishna · March 8, 2019 at 2:38 PM
Awesome Tutorial for Java . thanku It is very Helpfull for Us.