Table of Contents
hide
Ques. : Create two Activity pages in an Android App to Open another Activity from the First Activity by clicking the button using Intent concept and XML & Java files in Linear Layout View.
NB: To create two or more Activity Page in an Android App -
Open an Android application - Right click on Java option(below manifests
file) - New - Activity - Empty Views Activity - both the XML and Java
files created and appeared.
activity_main.xml
------------------
MainActivity.java
------------------
package com.rkm.myapplication;
import androidx.appcompat.app.AppCompatActivity;
import android.annotation.SuppressLint;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
public class MainActivity extends AppCompatActivity {
Button button1;
@SuppressLint("MissingInflatedId")
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
button1=(Button) findViewById(R.id.Button1);
button1.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
Intent intent1= new Intent(MainActivity.this, MainActivity2.class);
startActivity(intent1);
}
});
}
}
===============================================================================
activity_main2.xml
--------------------
MainActivity2.java
--------------------
package com.rkm.myapplication;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
public class MainActivity2 extends AppCompatActivity {
Button button2;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main2);
button2=(Button) findViewById(R.id.Button2);
button2.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
Intent intent2= new Intent(MainActivity2.this, MainActivity.class);
startActivity(intent2);
}
});
}
}
Output:
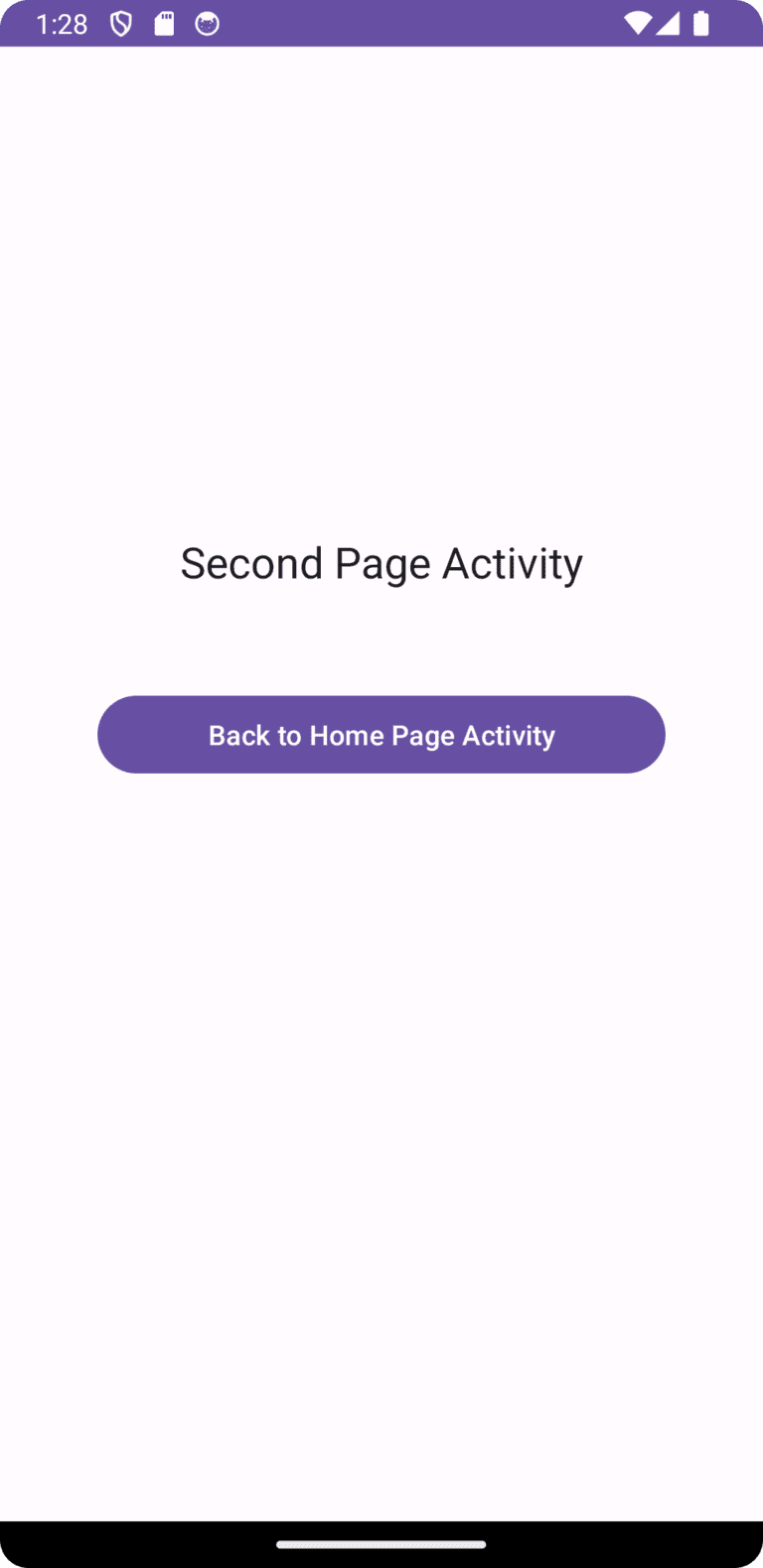
Ques. : Create an Activity page in an Android App to Play/Pause/Stop MP3 songs using Media Player by clicking the button using XML & Java files in Linear Layout View.
NB: First of all create Song list resource[Right click on 'res' folder in an
open android application - New - Android Resource Directory - Select 'Raw' from Resource type - ok - Copy mp3 files and paste in 'Raw' folder in 'res'
folder.]
activity_main.xml
--------------------
MainActivity.java
-------------------
package com.rkm.myapplication;
import androidx.appcompat.app.AppCompatActivity;
import android.annotation.SuppressLint;
import android.media.MediaPlayer;
import android.os.Bundle;
import android.view.View;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity
{
MediaPlayer Player;
int length;
@SuppressLint("MissingInflatedId")
@Override
protected void onCreate(Bundle savedInstanceState)
{
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
public void playAudio(View v)
{
if(Player==null)
{
Player=MediaPlayer.create(this,R.raw.song);
Player.start();
}
}
public void pauseAudio(View v)
{
if (Player != null)
{
Player.pause();
length=Player.getCurrentPosition();
}
}
public void resumeAudio(View v)
{
if (Player != null)
{
Player.seekTo(length);
Player.start();
}
}
public void stopAudio(View v)
{
stopPlayer();
}
private void stopPlayer()
{
if(Player!=null)
{
Player.release();
Player=null;
Toast.makeText(this, "Media Player Stopped", Toast.LENGTH_SHORT).show();
}
}
@Override
protected void onStop()
{
super.onStop();
stopPlayer();
}
}
Output:
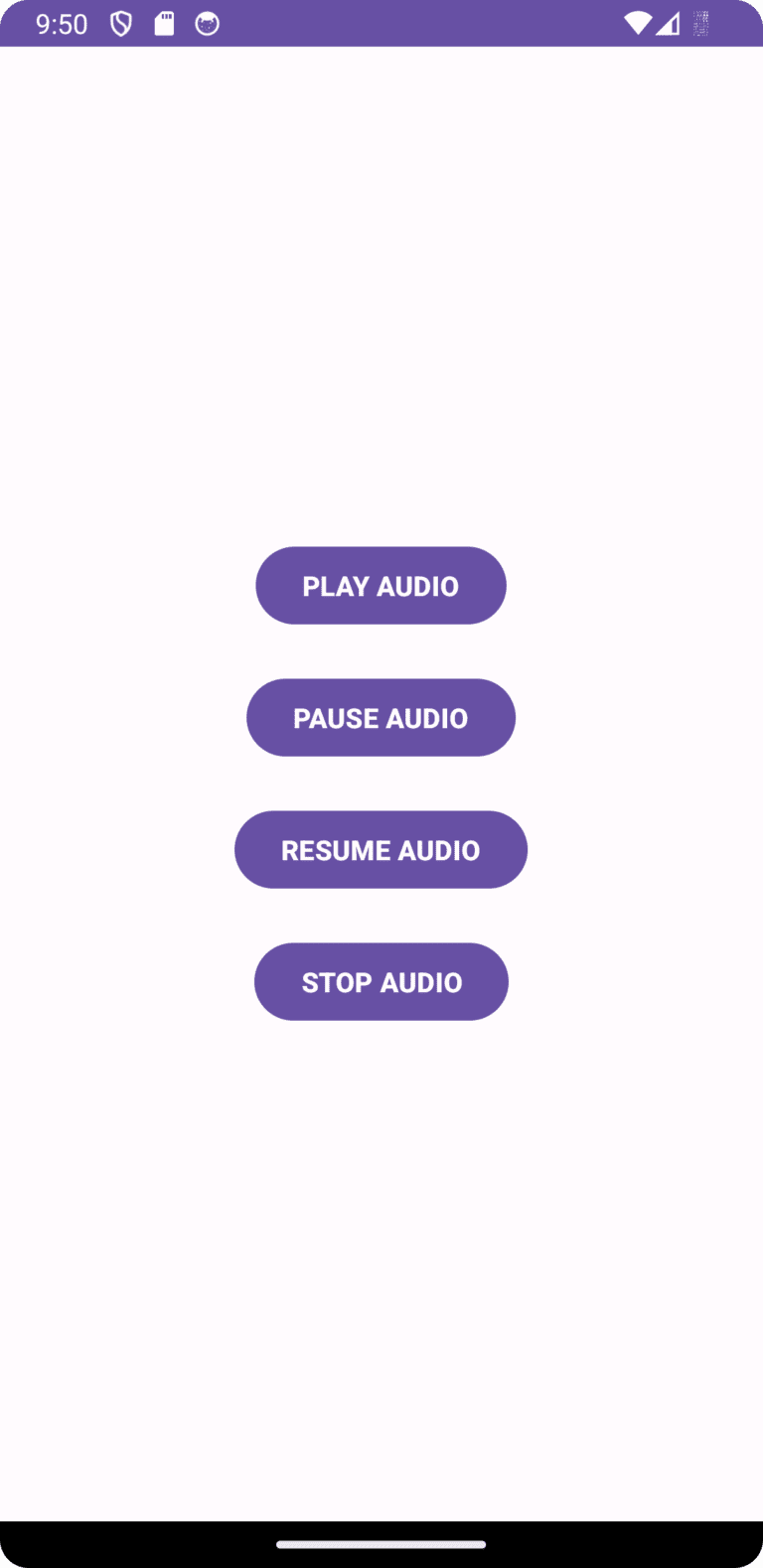
Ques. : An Android program to simply Play Video Files using XML and Android Java.
NB: First of all create Video list resource[Right click on 'res' folder in an
open android application - New - Android Resource Directory - Select 'Raw' from Resource type - ok - Copy Video files and paste in 'Raw' folder in 'res' folder.]
METHOD 1 [Using Static Video file Path]
activity_main.xml
--------------------
MainActivity.java
--------------------
package com.rkm.myapplication;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.widget.VideoView;
public class MainActivity extends AppCompatActivity
{
@Override
protected void onCreate(Bundle savedInstanceState)
{
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
VideoView videoview= findViewById(R.id.VideoPlayer);
String videoPath= "android.resource://" + getPackageName()+ "/raw/video1"; //put only video file name(video1) not extension.
videoview.setVideoPath(videoPath);
videoview.start();
}
}
METHOD 2 [Using URI Video file Path]
activity_main.xml
--------------------
"Code is similar as above."
MainActivity.java
--------------------
package com.rkm.myapplication;
import androidx.appcompat.app.AppCompatActivity;
import android.net.Uri;
import android.os.Bundle;
import android.widget.VideoView;
public class MainActivity extends AppCompatActivity
{
@Override
protected void onCreate(Bundle savedInstanceState)
{
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
VideoView videoview= findViewById(R.id.VideoPlayer);
String videoPath= "android.resource://" + getPackageName()+ "/raw/video1"; //put only video file name(video1) not extension.
Uri videoURi= Uri.parse(videoPath);
videoview.setVideoURI(videoURi);
videoview.start();
}
}
Output:
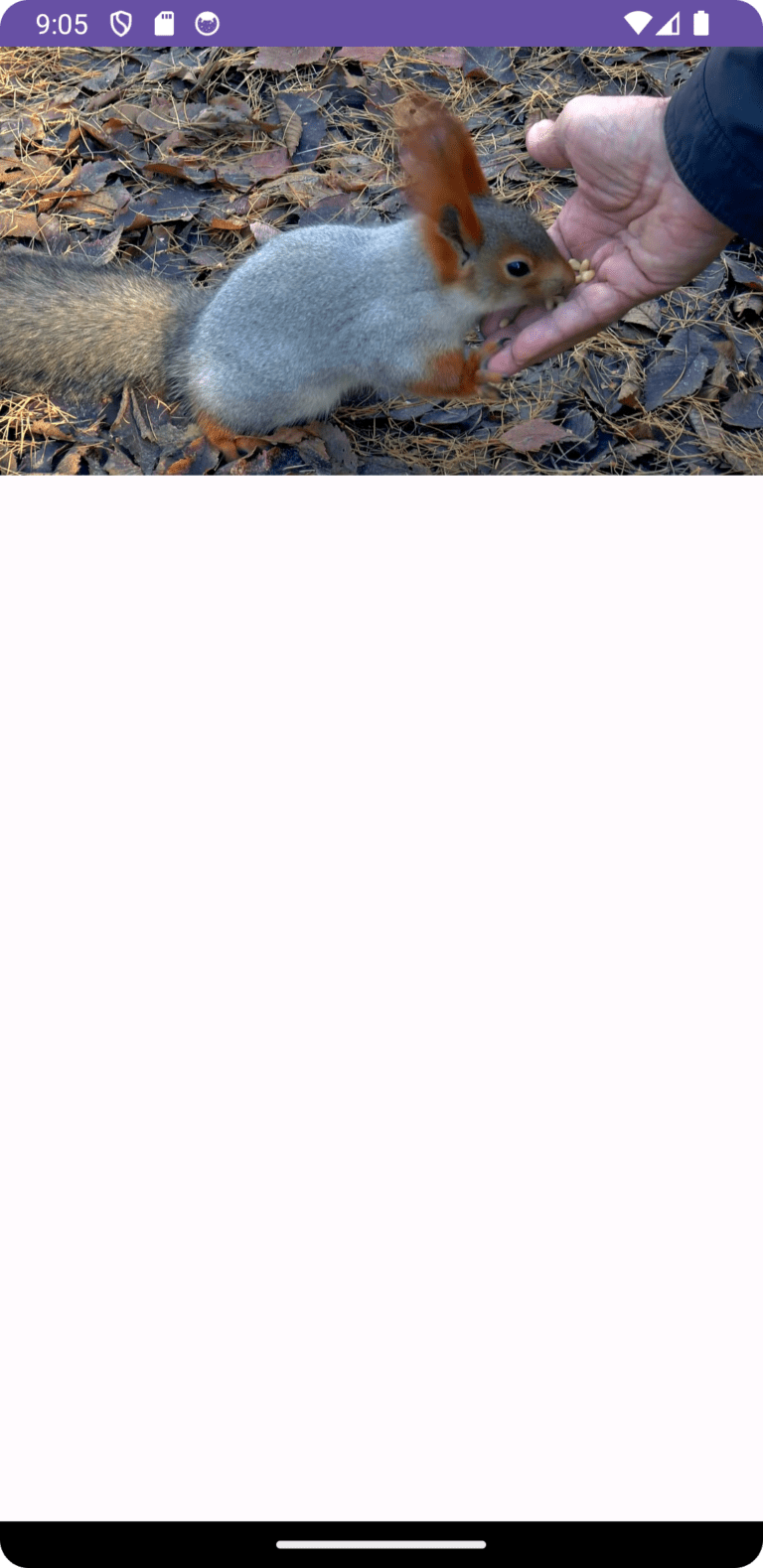
Ques. : An Android program to Play Video Files with Media Buttons at the bottom of the Apps Page using XML and Android Java.
NB: First of all create Video list resource[Right click on 'res' folder in an
open android application - New - Android Resource Directory - Select 'Raw' from Resource type - ok - Copy Video files and paste in 'Raw' folder in 'res' folder.]
activity_main.xml
--------------------
MainActivity.java
--------------------
package com.rkm.myapplication;
import androidx.appcompat.app.AppCompatActivity;
import android.net.Uri;
import android.os.Bundle;
import android.widget.MediaController;
import android.widget.VideoView;
public class MainActivity extends AppCompatActivity
{
@Override
protected void onCreate(Bundle savedInstanceState)
{
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
VideoView videoview= findViewById(R.id.VideoPlayer);
String videoPath= "android.resource://" + getPackageName()+ "/raw/video1"; //put only video file name(video1) not extension.
Uri videoURi= Uri.parse(videoPath);
videoview.setVideoURI(videoURi);
videoview.start();
MediaController mdcontroller= new MediaController(this);
videoview.setMediaController(mdcontroller);
mdcontroller.setAnchorView(videoview);
}
}
Output:
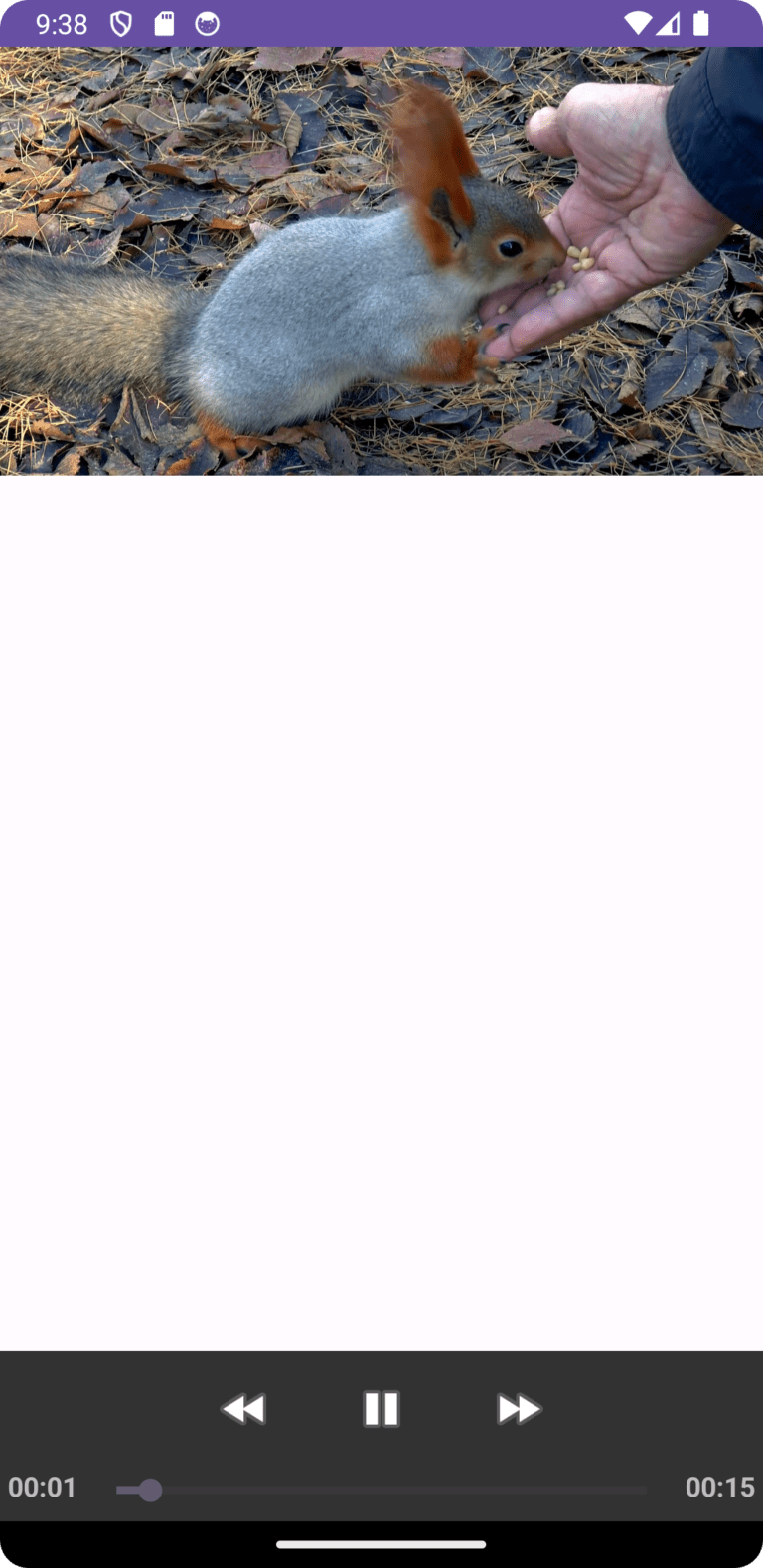
Ques. : An Android program to Play Video Files with Media Buttons just below the Video Player using XML and Android Java.
NB: First of all create Video list resource[Right click on 'res' folder in an
open android application - New - Android Resource Directory - Select 'Raw' from
Resource type - ok - Copy Video files and paste in 'Raw' folder in 'res' folder.]
activity_main.xml
--------------------
MainActivity.java
--------------------
package com.rkm.myapplication;
import androidx.appcompat.app.AppCompatActivity;
import android.net.Uri;
import android.os.Bundle;
import android.widget.MediaController;
import android.widget.VideoView;
public class MainActivity extends AppCompatActivity
{
@Override
protected void onCreate(Bundle savedInstanceState)
{
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
VideoView videoview= findViewById(R.id.VideoPlayer);
String videoPath= "android.resource://" + getPackageName()+ "/raw/video1"; //put only video file name(video1) not extension.
Uri videoURi= Uri.parse(videoPath);
videoview.setVideoURI(videoURi);
videoview.start();
MediaController mdcontroller= new MediaController(this);
videoview.setMediaController(mdcontroller);
mdcontroller.setAnchorView(videoview);
}
}
Output:
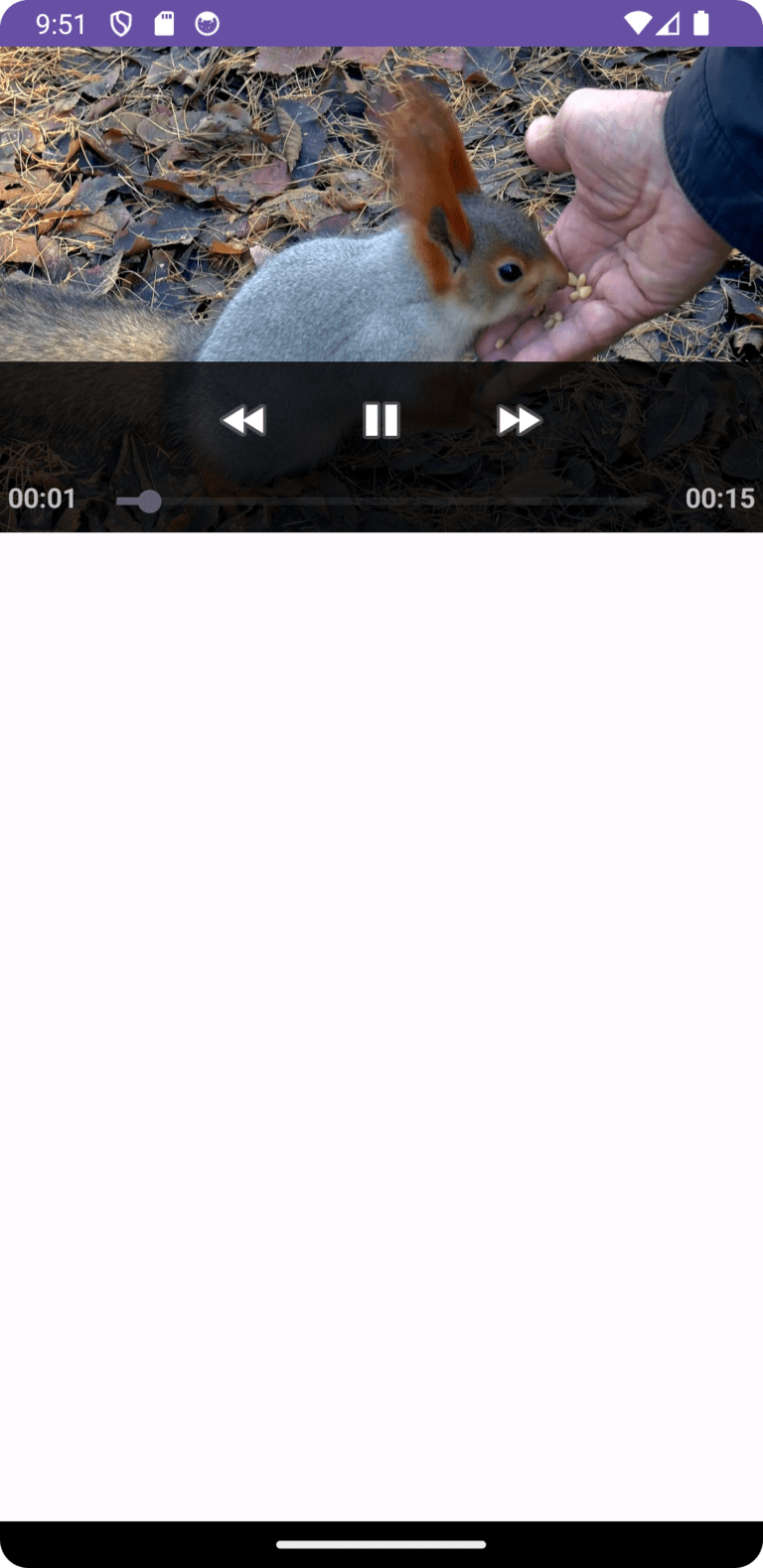
Ques. : An Android program to Play an Online Server or Internet Video Files with Media Buttons just below the Video Player using XML and Android Java.
NB: First set the Internet permission sinlge line code in AndroidManifests.xml file as below -
AndroidManifest.xml
---------------------
activity_main.xml
--------------------
MainActivity.java
--------------------
package com.rkm.myapplication;
import androidx.appcompat.app.AppCompatActivity;
import android.net.Uri;
import android.os.Bundle;
import android.widget.MediaController;
import android.widget.VideoView;
public class MainActivity extends AppCompatActivity
{
@Override
protected void onCreate(Bundle savedInstanceState)
{
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
VideoView videoview= findViewById(R.id.VideoPlayer);
String onlinePath="url of video file with extension";
Uri onlineVideoURi= Uri.parse(onlinePath);
videoview.setVideoURI(onlineVideoURi);
videoview.start();
MediaController mdcontroller= new MediaController(this);
videoview.setMediaController(mdcontroller);
mdcontroller.setAnchorView(videoview);
}
}
Output:
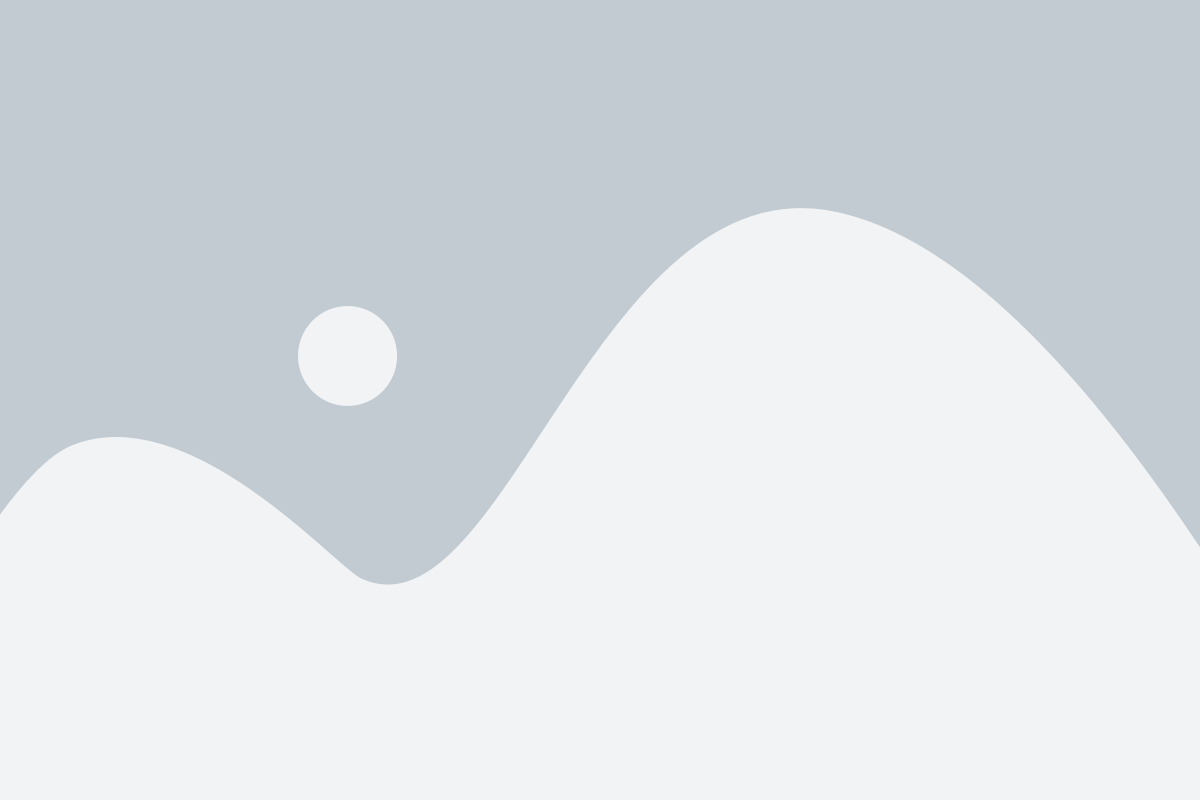
Ques. : An Android program to display different types of Messages using Toast.
activity_main.xml
--------------------
MainActivity.java
---------------------
package com.rkm.myapplication;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity
{
@Override
protected void onCreate(Bundle savedInstanceState)
{
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
public void ShowMessage(View v)
{
//Syntax: Toast.makeText(context, text, duration);
//(i) You can use getApplicationContext() or getActivity() or MainActivity.this or this(if Activity Name is MainActivity)
//Toast.makeText(MainActivity.this,"your message", Toast.LENGTH_LONG).show(); OR
//Toast.makeText(getApplicationContext(),"your message",Toast.LENGTH_SHORT).show();//Toast delay for 2000 ms predefined
//(ii) Inside Fragments (onCreateView)
//Toast.makeText(getActivity(), "your message" , Toast.LENGTH_LONG).show(); //Toast delay for 3500 ms predefined
//(iii) Inside Classes (onCreate)
//Toast.makeText(myClassName.this, "your message" , Toast.LENGTH_LONG).show();
Toast.makeText(this,"Toast Message Format1", Toast.LENGTH_LONG).show();
}
}
Output:
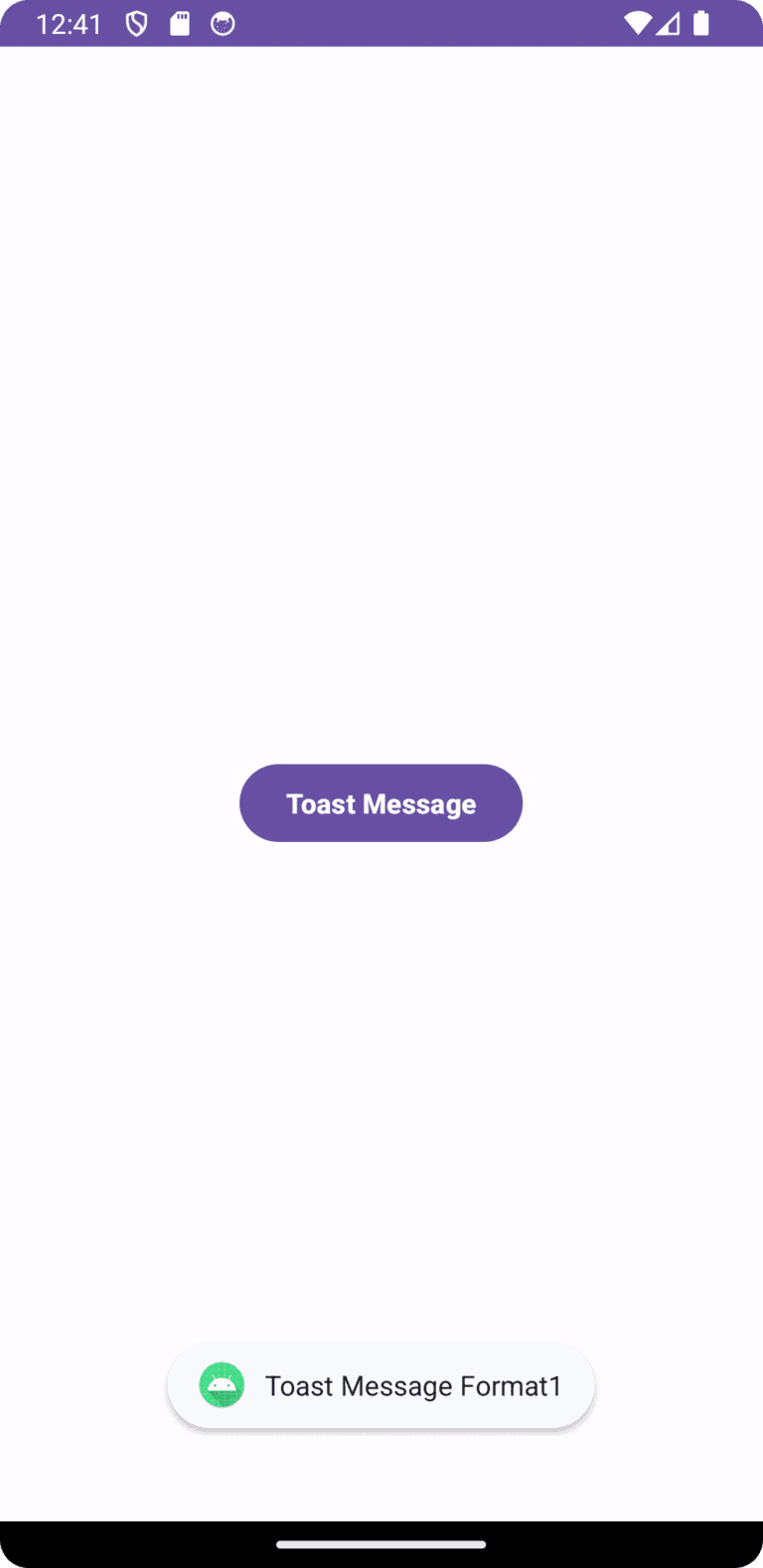
0 Comments